Learn the Basics of Python Programming: A Comprehensive Guide for Beginners

4.3 out of 5
Language | : | English |
File size | : | 939 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 87 pages |
Lending | : | Enabled |
Table of Contents
- Installation
- Basic Syntax
- Data Types
- Operators
- Control Flow
- Functions
- Modules
- Object-Oriented Programming
- Resources
Python is a versatile and powerful programming language that is widely used for a variety of applications, including web development, data analysis, and machine learning. If you're new to programming, Python is a great language to start with because it is relatively easy to learn and use.
In this guide, we'll cover the basics of Python programming, including installation, basic syntax, data types, operators, control flow, functions, modules, and object-oriented programming. By the end of this guide, you'll have a solid foundation in Python programming and be ready to start building your own programs.
Installation
To install Python on your computer, visit the official Python website and download the latest version of the Python interpreter for your operating system. Once you have downloaded the installer, follow the instructions to install Python on your computer.
Once Python is installed, you can open a terminal window and type the following command to verify that Python is installed correctly:
python --version
This command should output the version of Python that is installed on your computer.
Basic Syntax
Python uses a simple and straightforward syntax that makes it easy to read and write code. The following are some of the basic syntax rules of Python:
- Indentation is significant in Python. Code blocks are indented with four spaces or one tab.
- Statements end with a newline character.
- Comments start with a hash symbol (#).
- Variables are assigned values using the assignment operator (=).
Here is an example of a simple Python program:
# This is a Python program print("Hello, world!")
When you run this program, it will output the following message to the console:
Hello, world!
Data Types
Python has a variety of built-in data types, including:
- Integers (int)
- Floats (float)
- Strings (str)
- Lists (list)
- Tuples (tuple)
- Dictionaries (dict)
You can check the data type of a variable using the type()
function.
>>> x = 10 >>> type(x)
Operators
Python provides a variety of operators, including:
- Arithmetic operators (+, -, *, /, //, %)
- Comparison operators (<, >, <=, >=, ==, !=)
- Logical operators (and, or, not)
Operators can be used to perform a variety of operations on variables and data types.
Control Flow
Control flow statements allow you to control the flow of execution of your program. The following are some of the most common control flow statements in Python:
if
statementselif
statementselse
statementsfor
loopswhile
loops
Control flow statements can be used to perform a variety of tasks, such as making decisions, iterating over data, and repeating code.
Functions
Functions are reusable blocks of code that can be called from anywhere in your program. The following is the syntax for defining a function in Python:
def function_name(parameters): """Function documentation""" # Function body
Functions can be used to perform a variety of tasks, such as performing calculations, manipulating data, and interacting with the operating system.
Modules
Modules are self-contained units of code that can be imported into your program. The following is the syntax for importing a module in Python:
import module_name
Modules can be used to organize your code and share code between different programs.
Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that emphasizes the use of objects and classes to organize code. In OOP, objects are instances of classes that define their structure and behavior.
The following is an example of a simple Python class:
class Person: def __init__(self, name, age): self.name = name self.age = age
def get_name(self): return self.name
def get_age(self): return self.age
This class defines a Person
object that has two attributes, name
and age
. The __init__
method is the constructor for the class, and it is called when a new object is created. The get_name
and get_age
methods are getter methods that return the values of the name
and age
attributes, respectively.
Resources
- Official Python website
- Python documentation
- Python tutorial
- Python course
4.3 out of 5
Language | : | English |
File size | : | 939 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 87 pages |
Lending | : | Enabled |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Book
Novel
Page
Chapter
Text
Story
Genre
Reader
Library
Paperback
E-book
Magazine
Newspaper
Paragraph
Sentence
Bookmark
Shelf
Glossary
Bibliography
Foreword
Preface
Synopsis
Annotation
Footnote
Manuscript
Scroll
Codex
Tome
Bestseller
Classics
Library card
Narrative
Biography
Autobiography
Memoir
Reference
Encyclopedia
Jay Speight
Jane Tabachnick
Jane L Rosen
J C Sharman
James Gee
James W Anderson
Larry K Collins
Joy Bauer
Janice A Maville
Jay Singh Sohal
Janina Struk
James R Payne
Jeffrey Flanagan
Nick Popaditch
Territory Supply
James Traub
Kenneth S Rogoff
Panos Kompatsiaris
Jason M Barr
Paul Harris
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
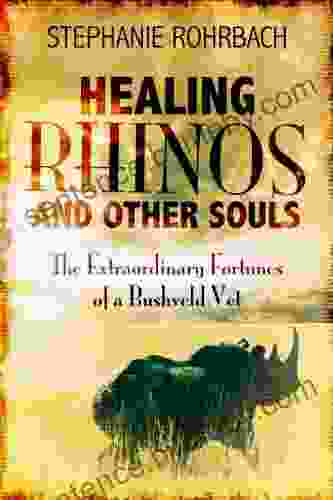

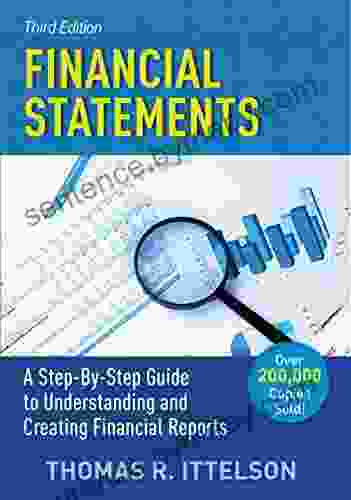

- Edgar Allan PoeFollow ·5.3k
- Clayton HayesFollow ·15.4k
- John MiltonFollow ·13.6k
- Preston SimmonsFollow ·10.7k
- August HayesFollow ·16.8k
- Chris ColemanFollow ·6.4k
- Ricky BellFollow ·16k
- Gerald ParkerFollow ·7.8k
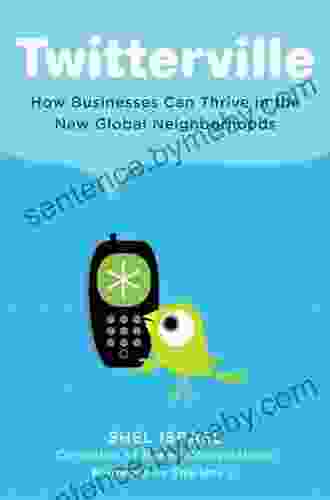

How Businesses Can Thrive In The New Global Neighborhoods
The world is becoming...
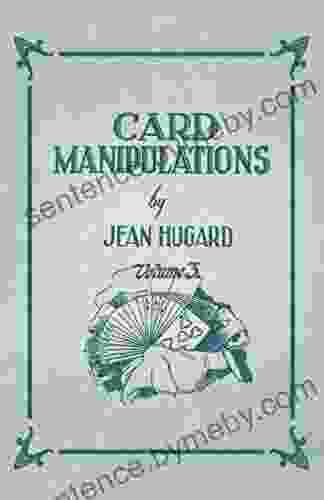

Card Manipulations Volume 1: A Masterclass in Deception...
Unveiling the...
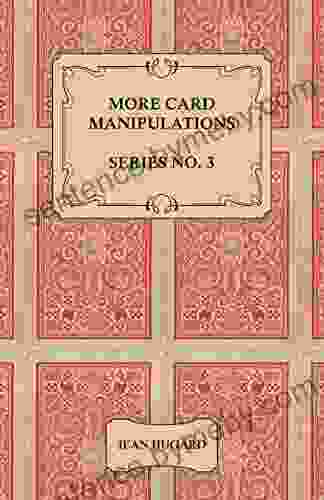

Unveil the Secrets of Card Manipulation: Dive into "More...
Step into the captivating world...
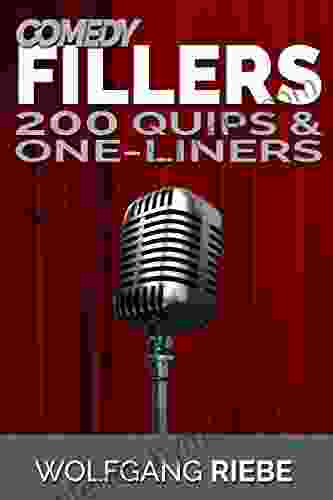

Comedy Fillers 200 Quips One Liners Jean Hugard
Unlock the Secrets of...
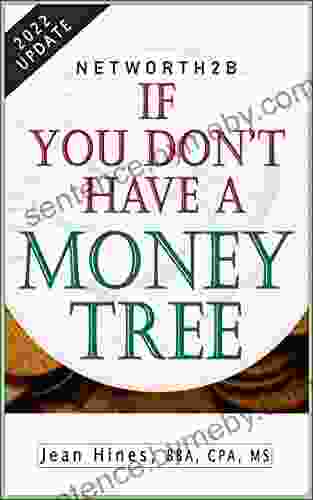

Unlock Financial Independence: A Comprehensive Guide to...
In a world where financial security seems...
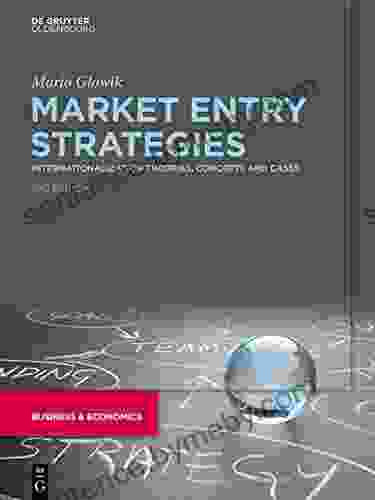

Unveiling Global Market Entry Strategies: A Comprehensive...
Global Market Entry Strategies:...
4.3 out of 5
Language | : | English |
File size | : | 939 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 87 pages |
Lending | : | Enabled |